Interested successful learning Python but don't cognize wherever to start? I'll locomotion you done the basics of the ever-popular programming connection step-by-step. In an hr oregon so, you'll spell from zero to penning existent code.
Set Up Your Development Environment
To commencement penning and moving Python programs locally connected your device, you indispensable person Python installed and an IDE (Integrated Development Environment) oregon substance editor, preferably a codification editor. First, let's instal Python. Go to the authoritative Python website. Go to Downloads. You should spot a fastener telling you to download the latest Python mentation (as of penning this, it's 3.13.3.) It volition besides amusement you antithetic operating systems for which you privation to download.
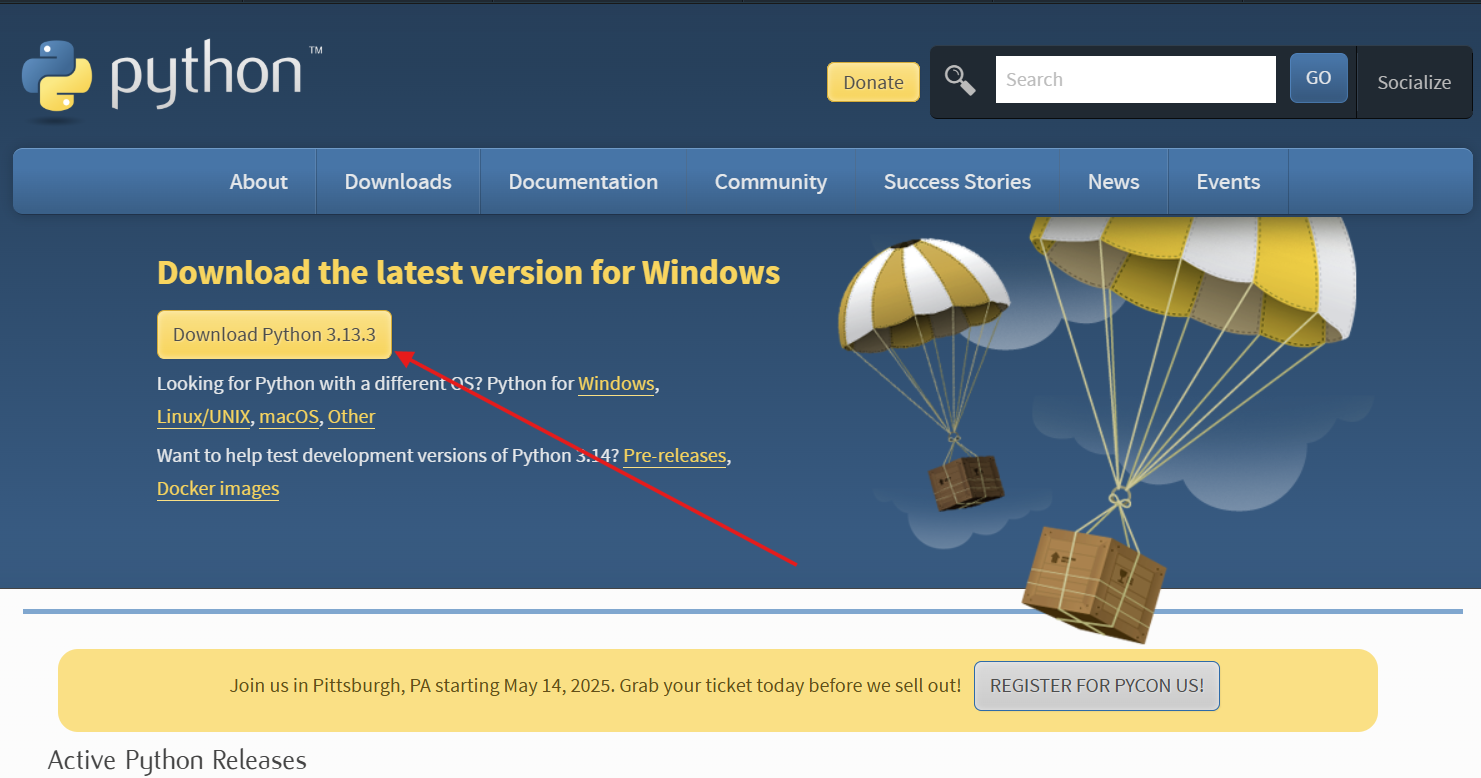
If you privation to install Python connected Windows, download the installer. Double-click to unfastened it. Follow the instructions and decorativeness the installation.
If you're utilizing a Linux organisation specified arsenic Ubuntu oregon macOS, astir apt Python has travel pre-installed with it. However, if it's not installed successful your distro, you tin either usage your distro's bundle manager to instal it oregon physique it from source. For macOS, you tin usage the Homebrew bundle manager oregon the official macOS installer.
After installing, you'll find a Python ammunition installed called IDLE connected your system. You tin commencement penning Python codification connected that. But for much features and flexibility, it's amended to spell with a bully codification exertion oregon an IDE. For this tutorial, I'll beryllium utilizing Visual Studio Code. You tin get it connected immoderate of the 3 operating systems.
If you're utilizing VS Code, 1 much happening you'll request is an hold called Code Runner. This isn't strictly required but volition marque your beingness easier. Go to the Extensions tab, and hunt for Code Runner. Install the extension. After that, you should spot a tally fastener astatine the apical close country of your editor.
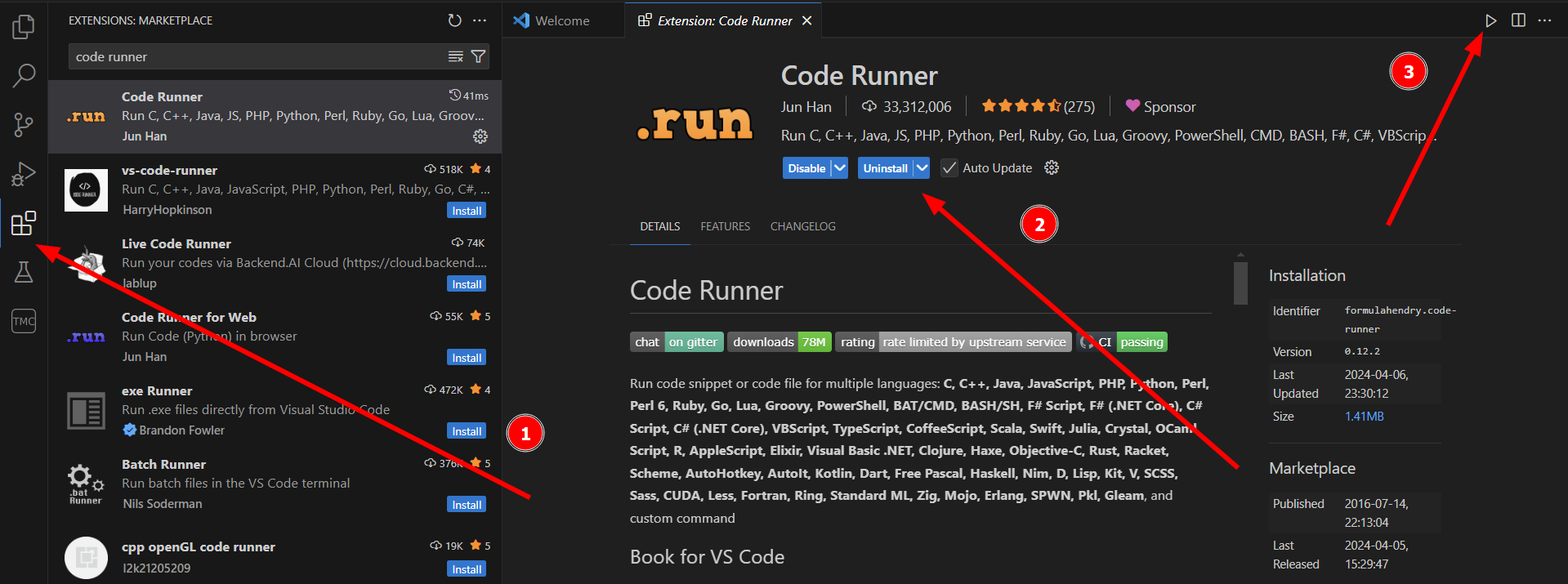
Now, each clip you privation to execute your code, you tin click connected that tally button. With that, you're acceptable to constitute immoderate code.
Write Your First Python Program
Create a record and sanction it "hello.py" without quotes. You tin usage different names, too, but adding the .py astatine the extremity is important. For our archetypal program, let's people a drawstring oregon substance successful the console, besides known arsenic the bid enactment interface. For that, we'll usage the print() relation successful Python. Write this successful your codification editor:
print("Hello, Python")
Now tally your code. It should people "Hello, Python" without the quotes connected the console.
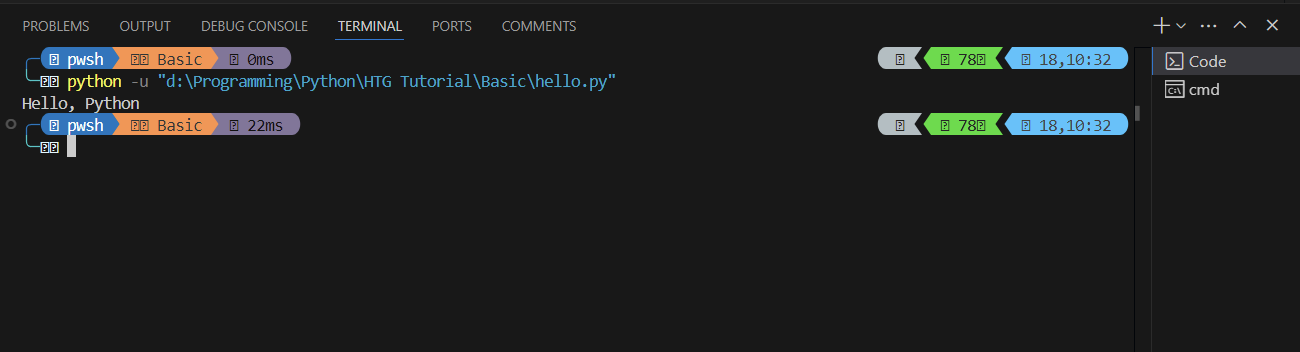
You tin constitute thing betwixt the quotes wrong the print() function, which volition beryllium displayed. We'll larn much astir functions later.
Comments are lines that are not executed erstwhile you tally your code. You tin usage comments to explicate codification segments truthful that erstwhile idiosyncratic other looks astatine your programme oregon you travel backmost to it aft a agelong time, you tin recognize what's going on. Another usage lawsuit for comments is erstwhile you don't privation to execute a line, you tin remark it out.
To remark retired a enactment successful Python, we usage the # symbol. Start immoderate enactment with that awesome and it volition beryllium treated arsenic a comment.
print("Hello, Python")
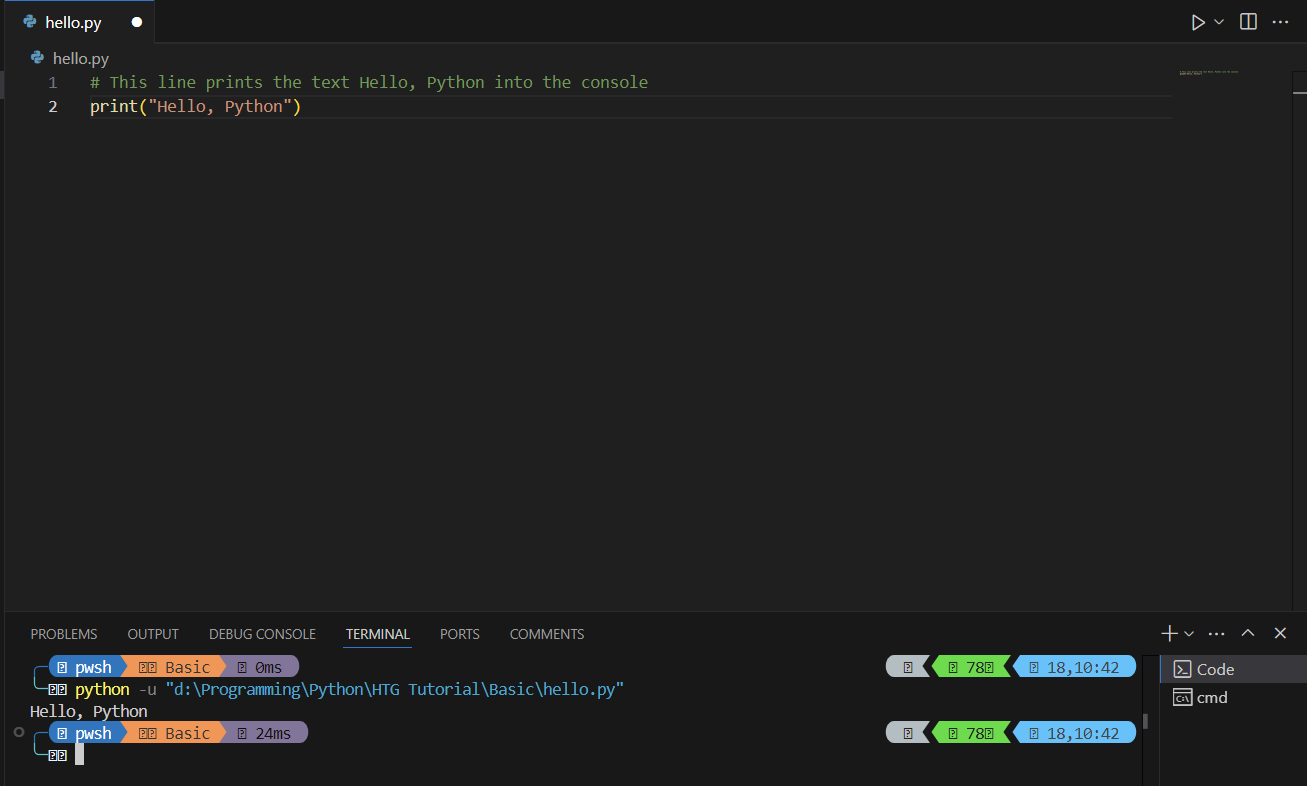
The archetypal enactment is simply a remark and won't beryllium picked up erstwhile executed. You tin adhd a remark connected the close broadside of your codification arsenic well.
print("Hello, Python") # This enactment prints the substance Hello, Python into the console
You tin adhd arsenic galore comments successful aggregate lines arsenic you want.
print("Hello, Python")
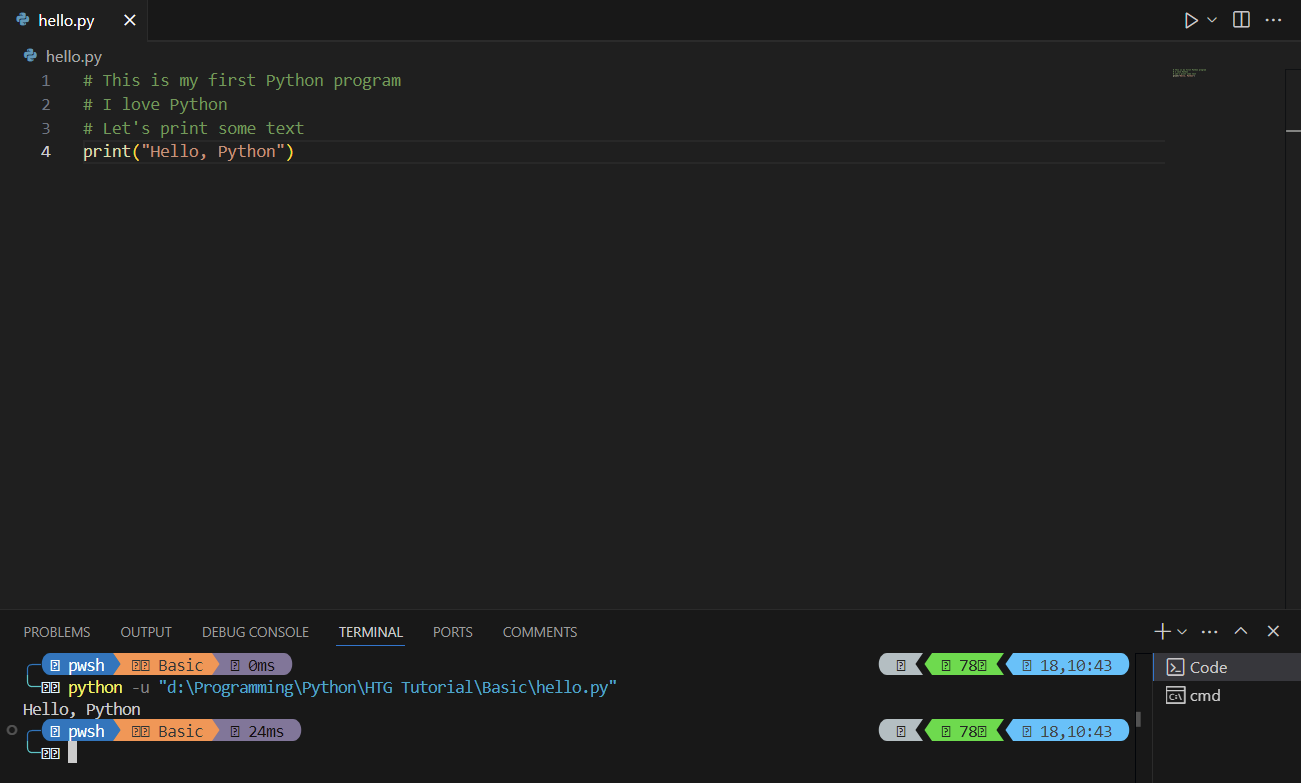
Another commonly utilized strategy for multiline comments is utilizing triple quotes. You tin bash the aforesaid arsenic supra with this code.
"""This is my archetypal Python programI emotion Python
Let's people immoderate text"""
print("Hello, Python")
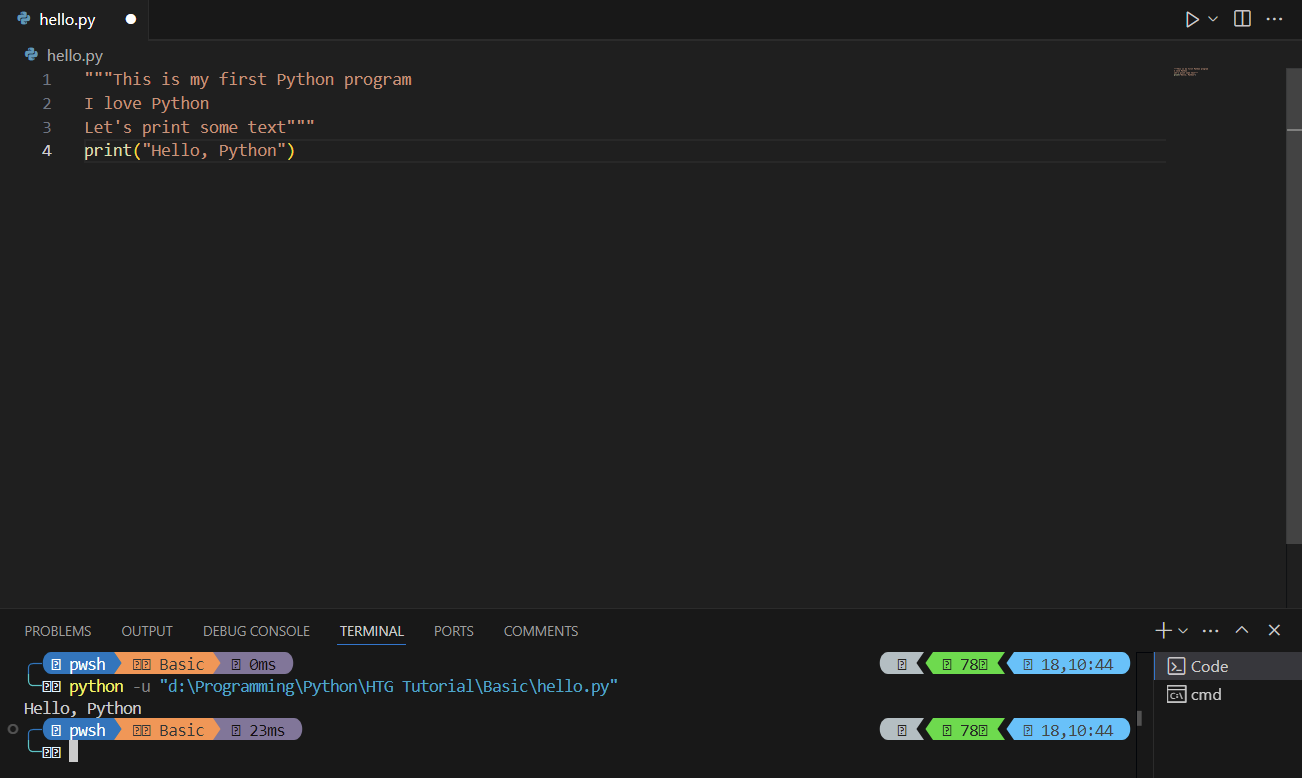
Store Data successful Variables
Variables are similar containers. They tin clasp values for you, specified arsenic text, numbers, and more. To delegate a worth to a variable, we travel this syntax:
a = 5Here, we're storing the worth 5 successful the adaptable "a". Likewise, we tin store strings.
a = "Python"A champion signifier for penning adaptable names is to beryllium descriptive truthful that you tin place what worth it's storing.
property = 18You tin people a variable's worth to the console.
sanction = "John"print(name)
This volition people "John" connected the console. Notice that successful the lawsuit of variables, we don't necessitate them to beryllium wrong quotes erstwhile printing. Variable names person immoderate rules to follow.
- They can't commencement with numbers. But you tin adhd numbers successful the middle.
- You can't person non-alphanumeric characters successful them.
- Both uppercase and lowercase letters are allowed.
- Starting with an underscore (_) is allowed.
- For longer names, you tin abstracted each connection utilizing an underscore.
- You can't usage definite reserved words (such arsenic class) for adaptable names.
Here are immoderate valid and invalid adaptable sanction examples:
name = "Alice"
age = 30
user_name = "alice123"
number1 = 42
_total = 100
1name = "Bob"
user-name = "bob"
total$ = 50
class = "math"
Learn Python's Data Types
In Python, everything is an object, and each entity has a information type. For this tutorial, I'll lone absorption connected a fewer basal information types that screen astir usage cases.
Integer
This is simply a numeric information type. These are full numbers without a decimal point. We usage the int keyword to correspond integer data.
property = 25year = 2025
Float
These are numbers with a decimal point. We correspond them with the interval keyword.
price = 19.99temperature = -3.5
String
These are substance enclosed successful quotes (single ' oregon treble " some work.) The keyword associated with strings is str.
sanction = "Alice"greeting = 'Hello, world!'
Boolean
This benignant of adaptable tin clasp lone 2 values: True and False.
is_logged_in = Truehas_permission = False
To spot what information benignant a adaptable is of, we usage the type() function.
print(type(name))print(type(price))
print(type(is_logged_in))
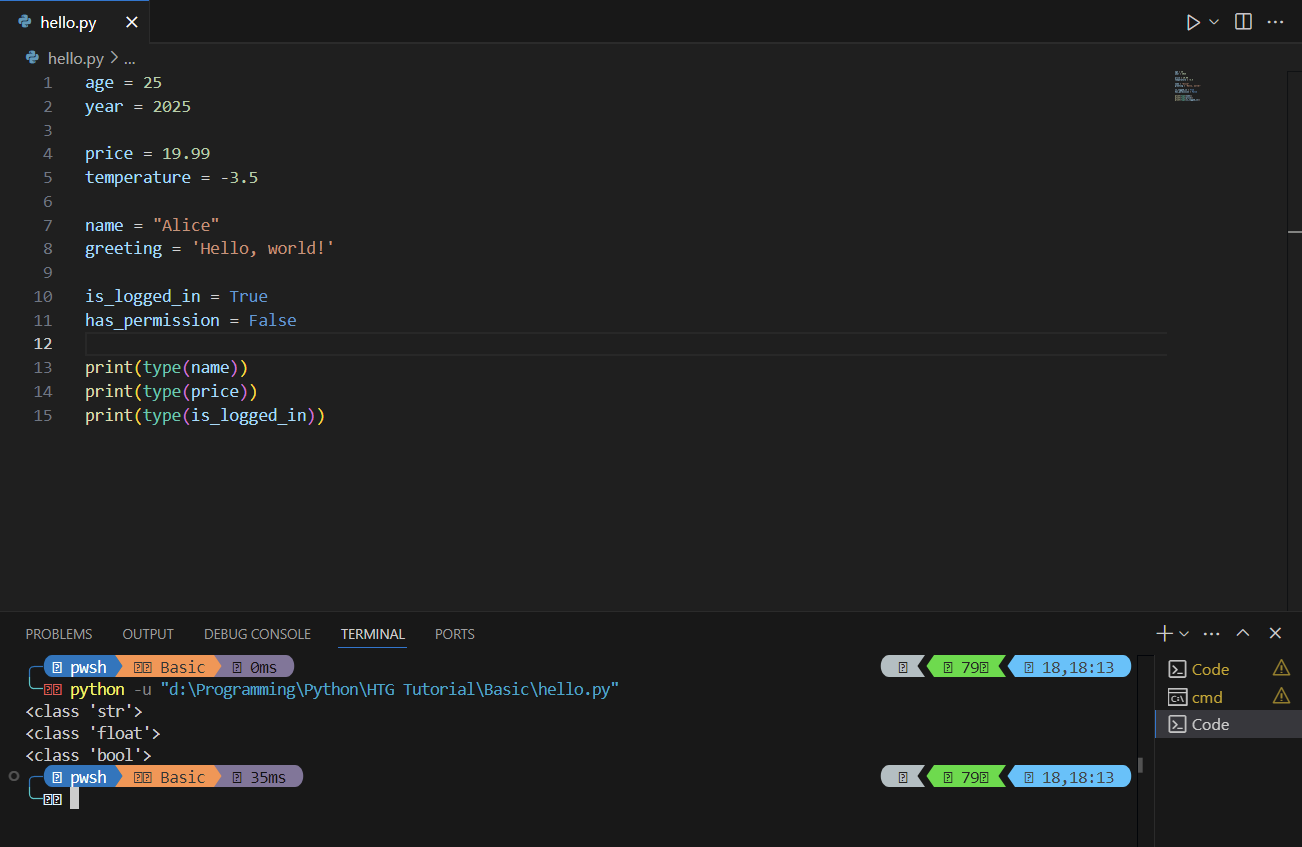
Convert Between Data Types (Typecasting)
Python has a mode to person 1 benignant of information to another. For example, turning a fig into a drawstring to people it, oregon converting idiosyncratic input (which is ever a string) into an integer for calculations. This is called typecasting. For that, we person antithetic functions:
int() |
Integer |
float() |
Float |
bool() |
Boolean |
str() |
String |
Here are immoderate examples:
age_str = "25"age = int(age_str)
print(age + 5)
score = 99
score_str = str(score)
print("Your people is " + score_str)
pi = 3.14
rounded = int(pi)
whole_number = 10
decimal_number = float(whole_number)
print(bool(0))
print(bool("hello"))
print(bool(""))
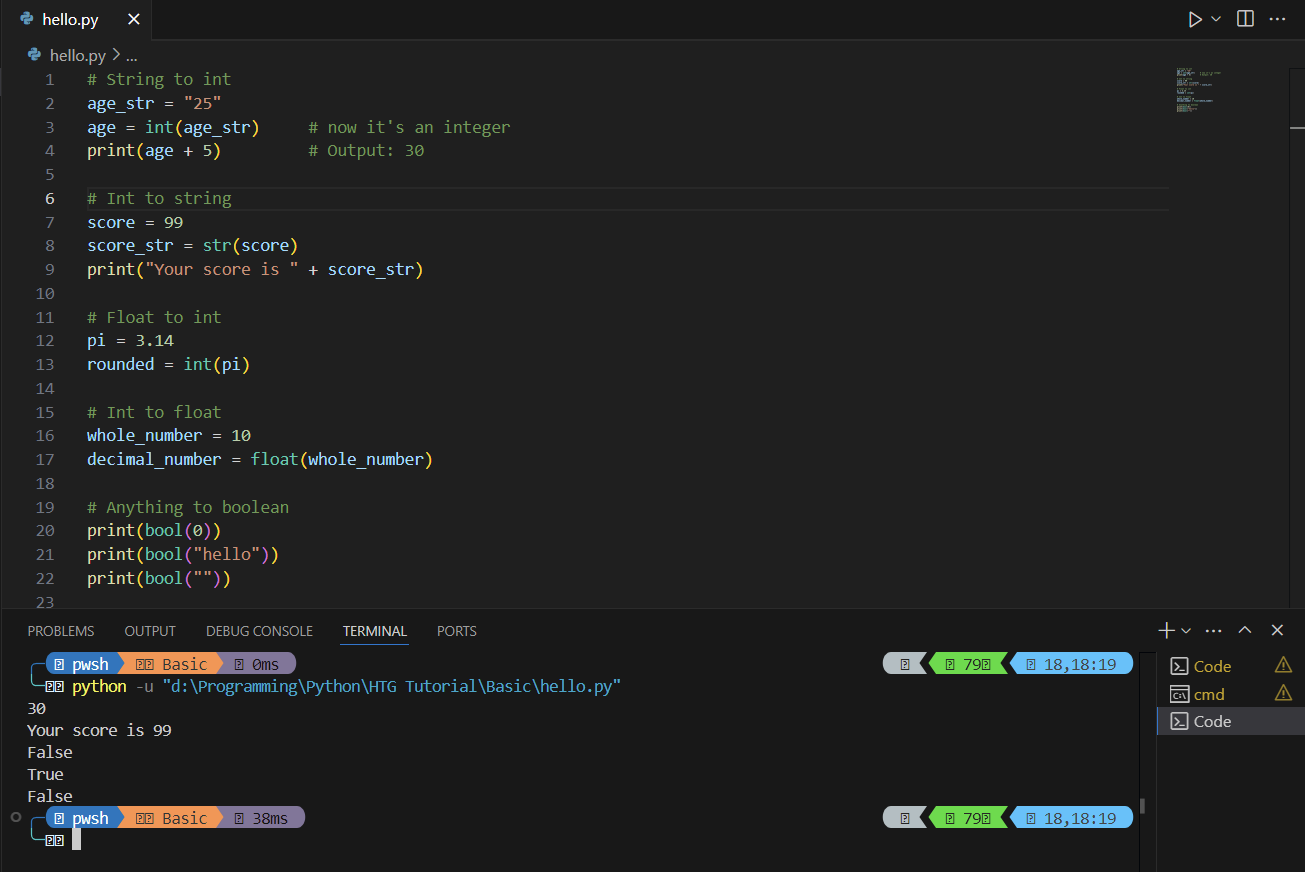
Take User Input
Until now, we person straight hardcoded values into variables. However, you tin marque your programs much interactive by taking input from the user. So, erstwhile you tally the program, it volition punctual you to participate something. After that, you tin bash immoderate with that value. To instrumentality idiosyncratic input, Python has the input() function. You tin besides store the idiosyncratic input successful a variable.
sanction = input()To marque the programme much understandable, you tin constitute a substance wrong the input function.
sanction = input("What is your name?")print("Hello", name)
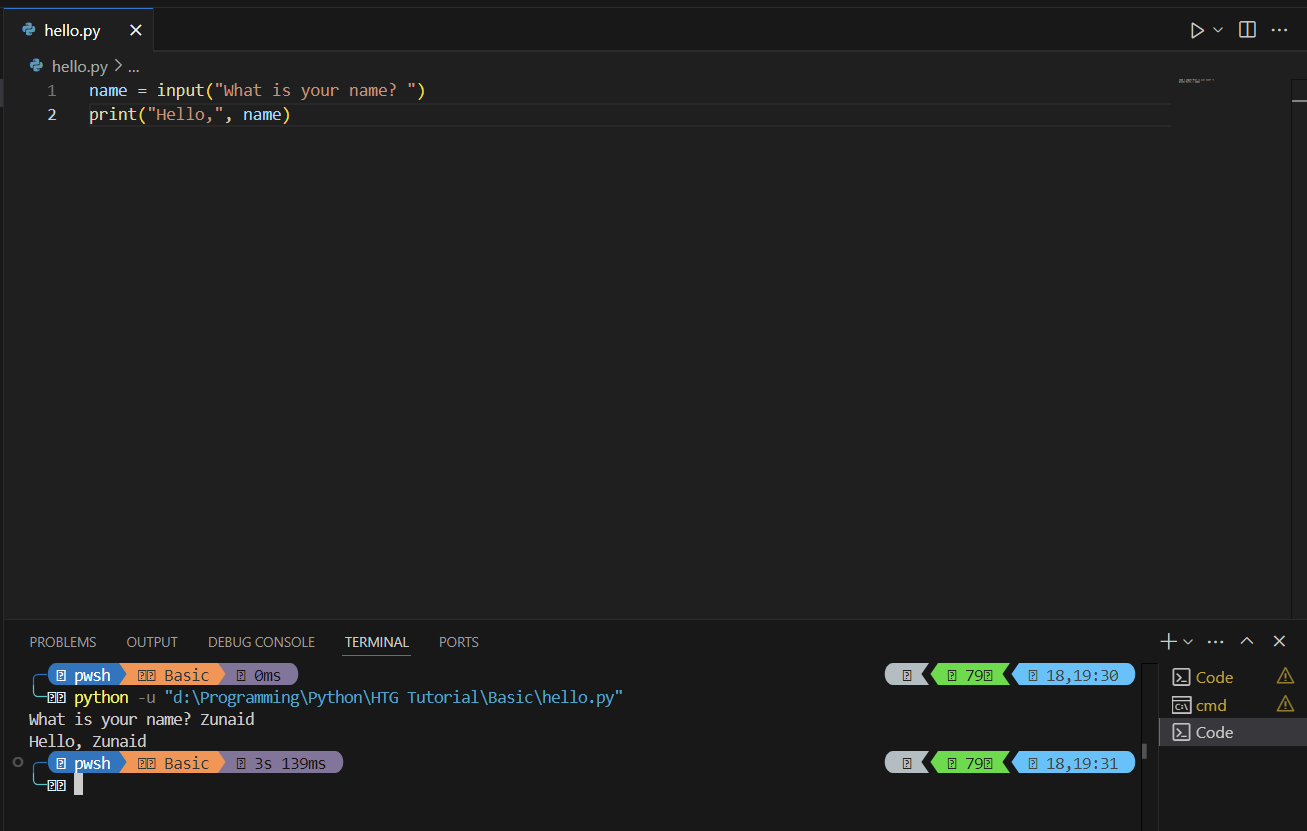
Know that inputs are ever taken arsenic strings. So, if you privation to bash calculations with them, you request to person them to different information type, specified arsenic an integer oregon float.
property = int(input("What is your age?"))print("In 5 years, you'll be", property + 5)
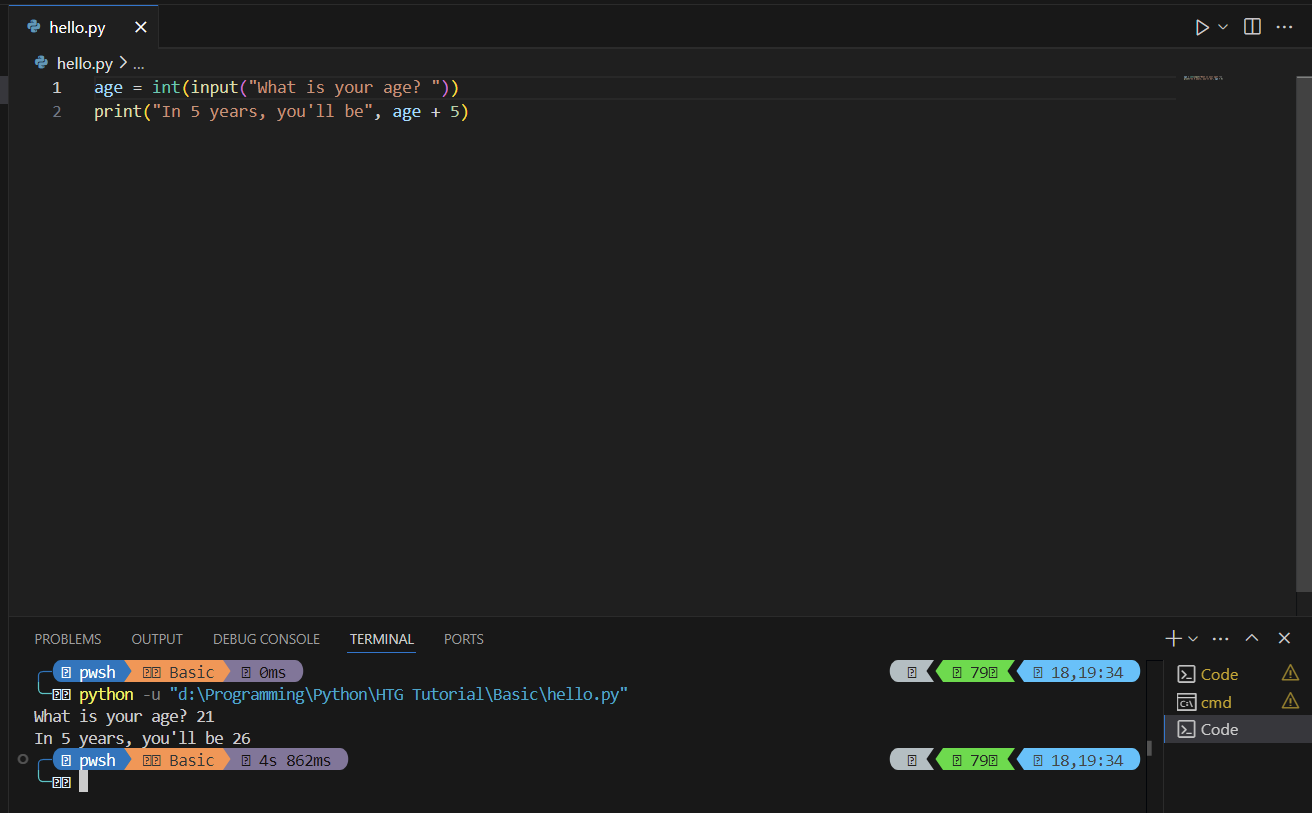
Do Math With Python
Python supports assorted types of mathematics. In this guide, we'll chiefly absorption connected arithmetic operations. You tin bash addition, subtraction, multiplication, division, modulus, and exponentiation successful Python.
x = 10y = 3
a = x + y
b = x - y
c = x * y
d = x / y
g = x // y
e = x % y
f = x ** y
print("Addition: ", a)
print("Subtraction: ", b)
print("Multiplication: ", c)
print("Division: ", d)
print("Floor Division: ", g)
print("Modulus: ", e)
print("Exponent: ", f)
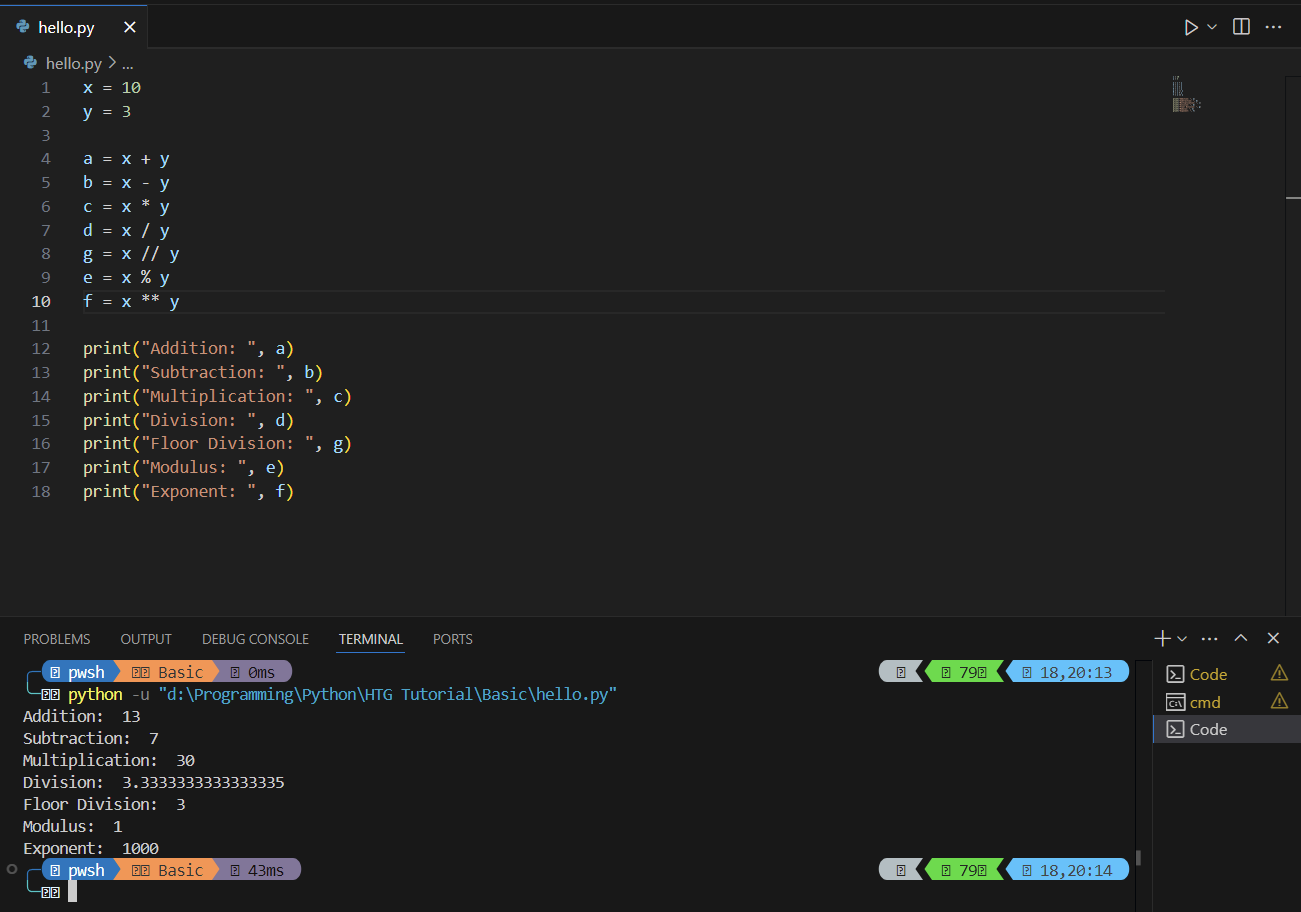
You tin bash overmuch much precocious calculations successful Python. The Python mathematics module has galore mathematical functions. However, that's not successful the scope of this guide. Feel escaped to research them.
Use Comparison Operators
Comparison operators fto you comparison values. The effect is either True oregon False. This is ace utile erstwhile you privation to marque decisions successful your code. There are six examination operators.
== |
Equal to |
!= |
Not adjacent to |
> |
Greater than |
< |
Less than |
>= |
Greater than oregon equal |
<= |
Less than oregon equal |
Here are immoderate examples:
a = 10b = 7
print(a > b)
print(a == b)
print(a < b)
print(a != b)
print(a >= b)
print(a <= b)
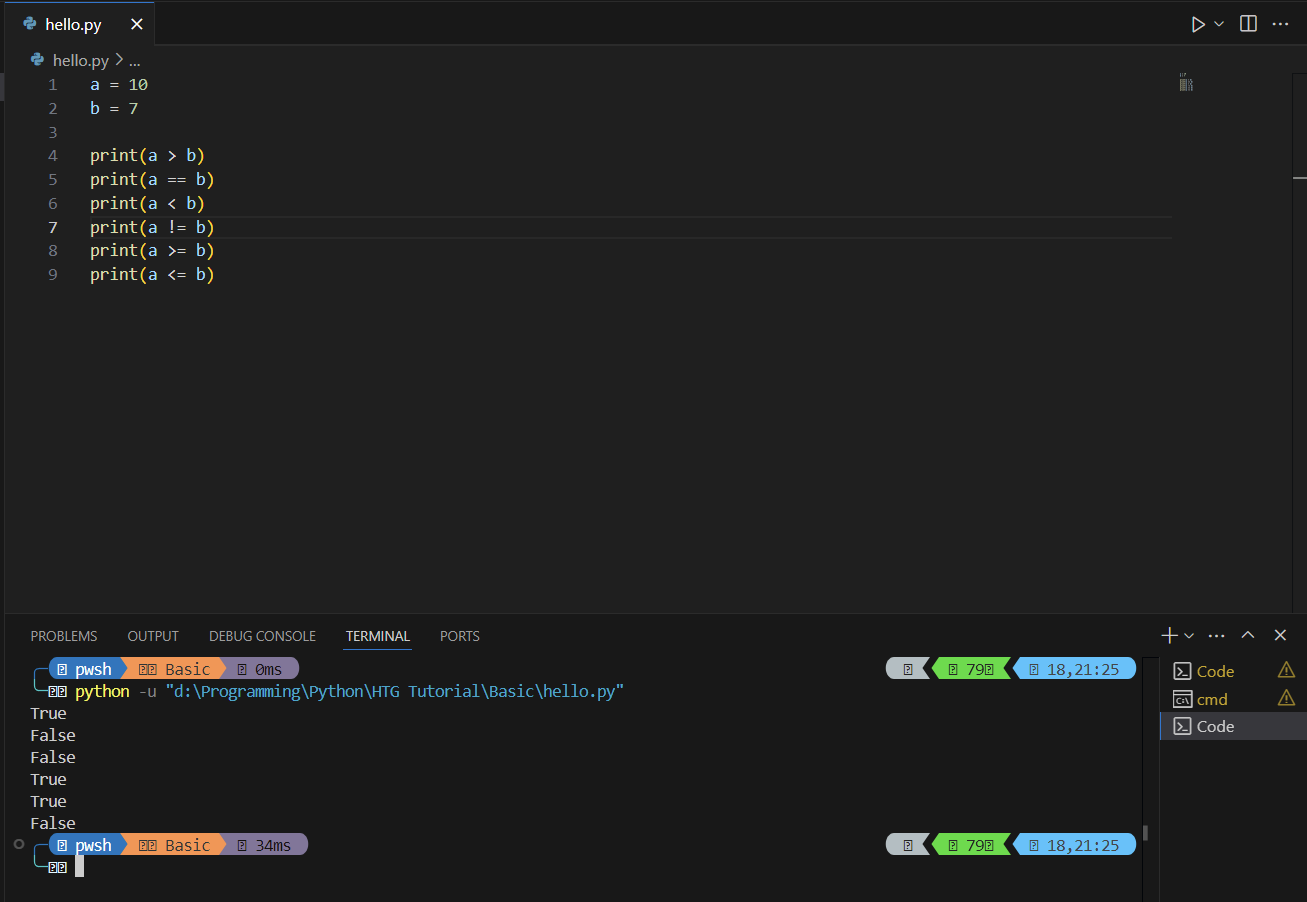
Apply Logical Operators
Logical operators assistance you harvester aggregate conditions and retrurns True oregon False based connected the conditions. There are 3 logical operators successful Python.
and |
True if some are true |
or |
True if astatine slightest 1 is true |
not |
Flips the result |
Some examples volition marque it clearer.
property = 17has_license = True
print(age >= 18 and has_license)
day = "Saturday"
print(day == "Saturday" or time == "Sunday")
is_logged_in = False
print(not is_logged_in)
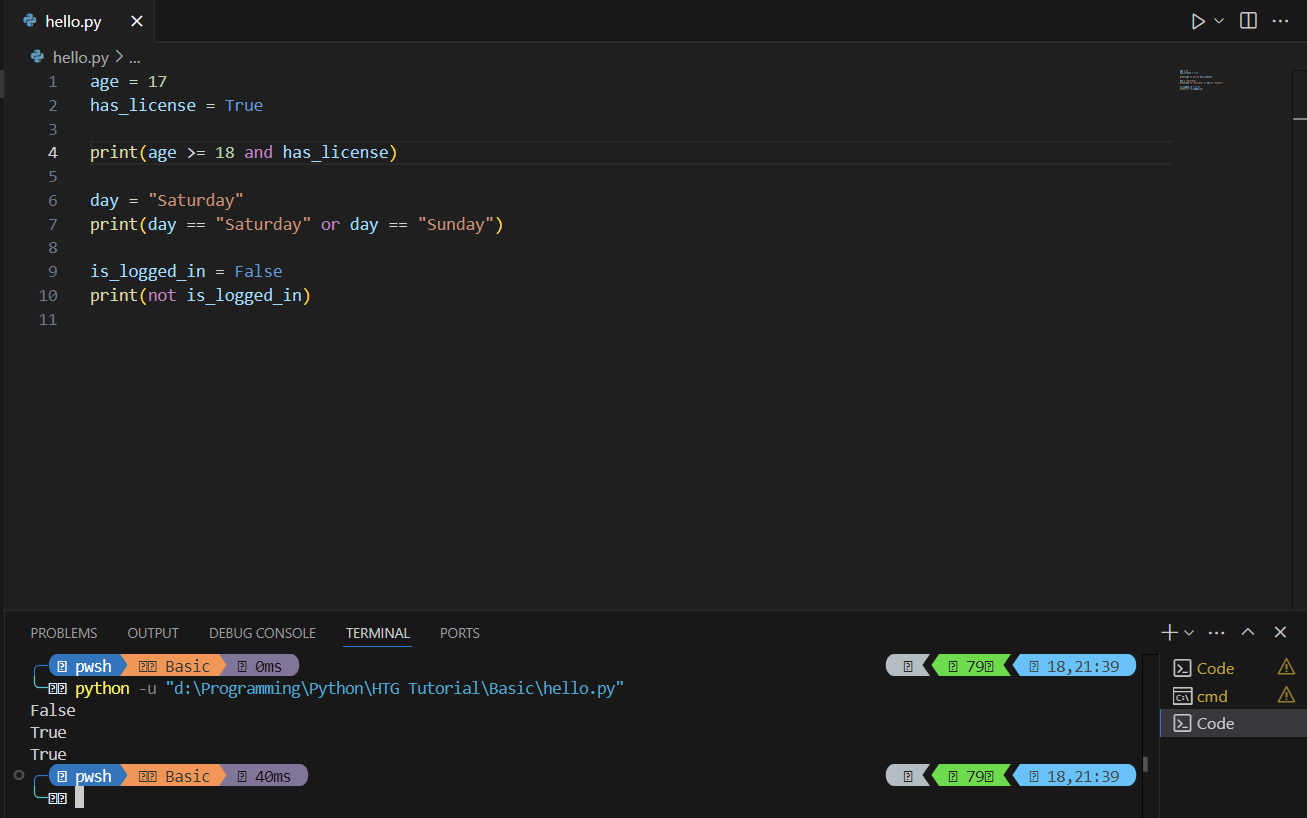
Write Conditional Statements
Now that you’ve learned astir examination and logical operators, you’re acceptable to usage them to marque decisions successful your codification utilizing conditional statements. Conditional statements fto your programme take what to bash based connected definite conditions. Just similar real-life decision-making. There are 3 situations for conditional statements.
if Statement
We usage if erstwhile you privation to tally immoderate codification lone if a information is true.
if condition:Here's an example:
property = 18if property >= 18:
print("You're an adult.")
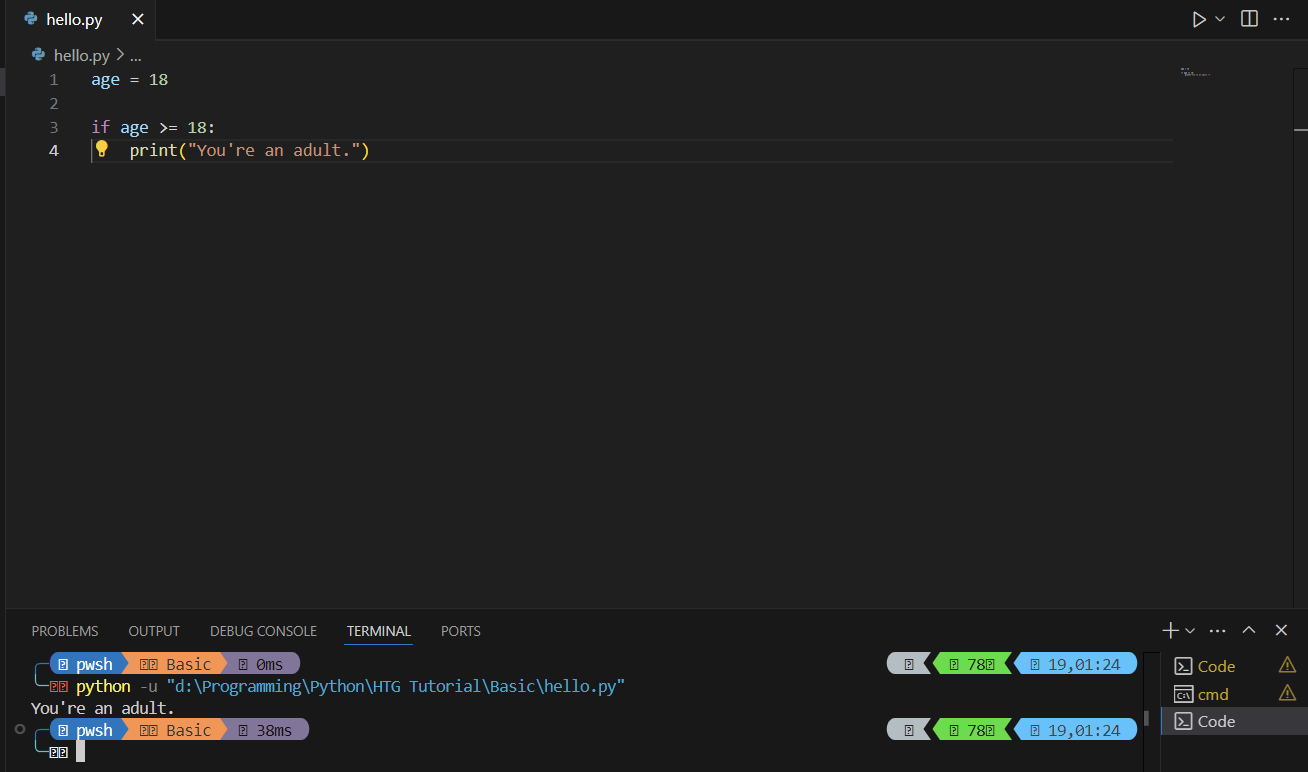
If the information is false, the codification wrong the if artifact is simply skipped.
In Python, indentation is crucial. Notice that the codification wrong the if artifact is indented. Python picks this up to recognize which enactment is portion of which block. Without due indentation, you'll get an error.
if-else Statement
We usage if-else erstwhile you privation to bash 1 happening if the information is true, and thing other if it's false.
if condition:else:
Example:
is_logged_in = Falseif is_logged_in:
print("Welcome back!")
else:
print("Please log in.")
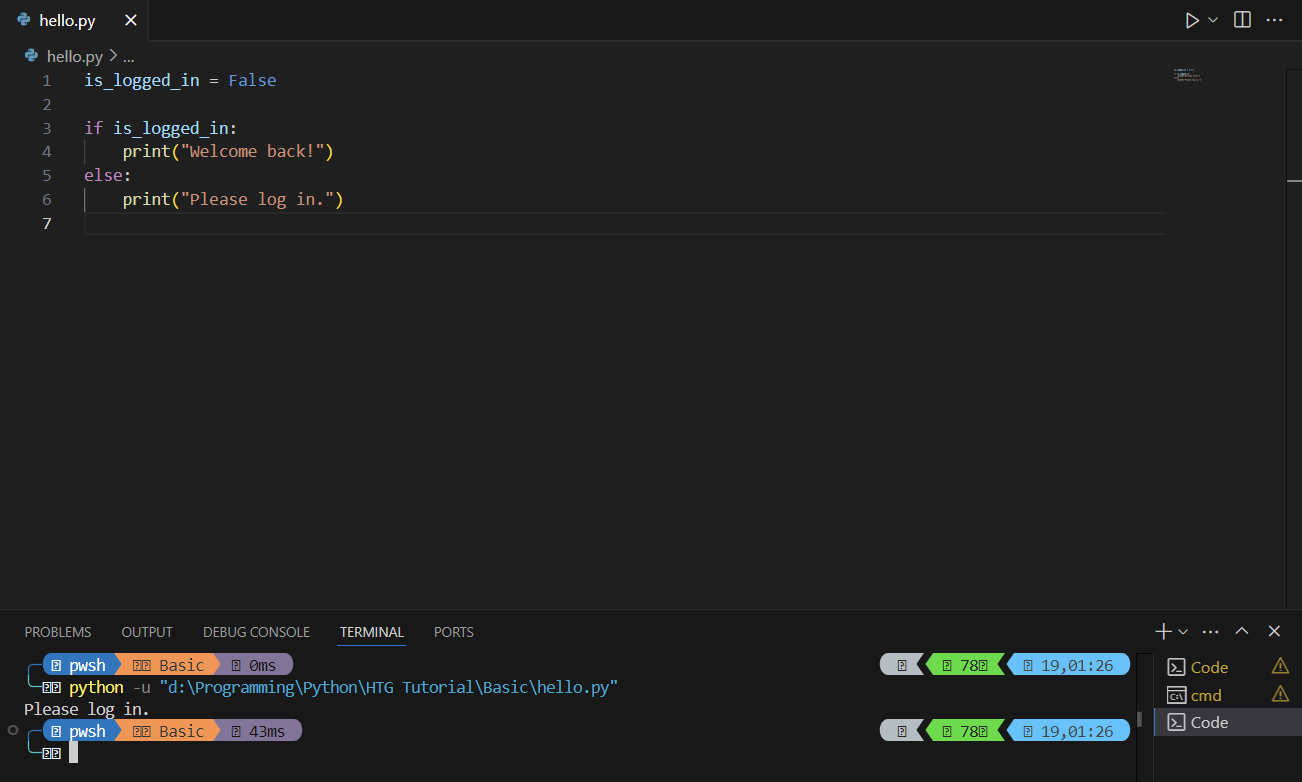
if-elif-else Statement
We usage if-elif-else erstwhile you person aggregate conditions to check, 1 aft the other.
if condition1:elif condition2:
else:
Let's spot an example.
people = 85if people >= 90:
print("Grade: A")
elif people >= 80:
print("Grade: B")
else:
print("Keep trying!")
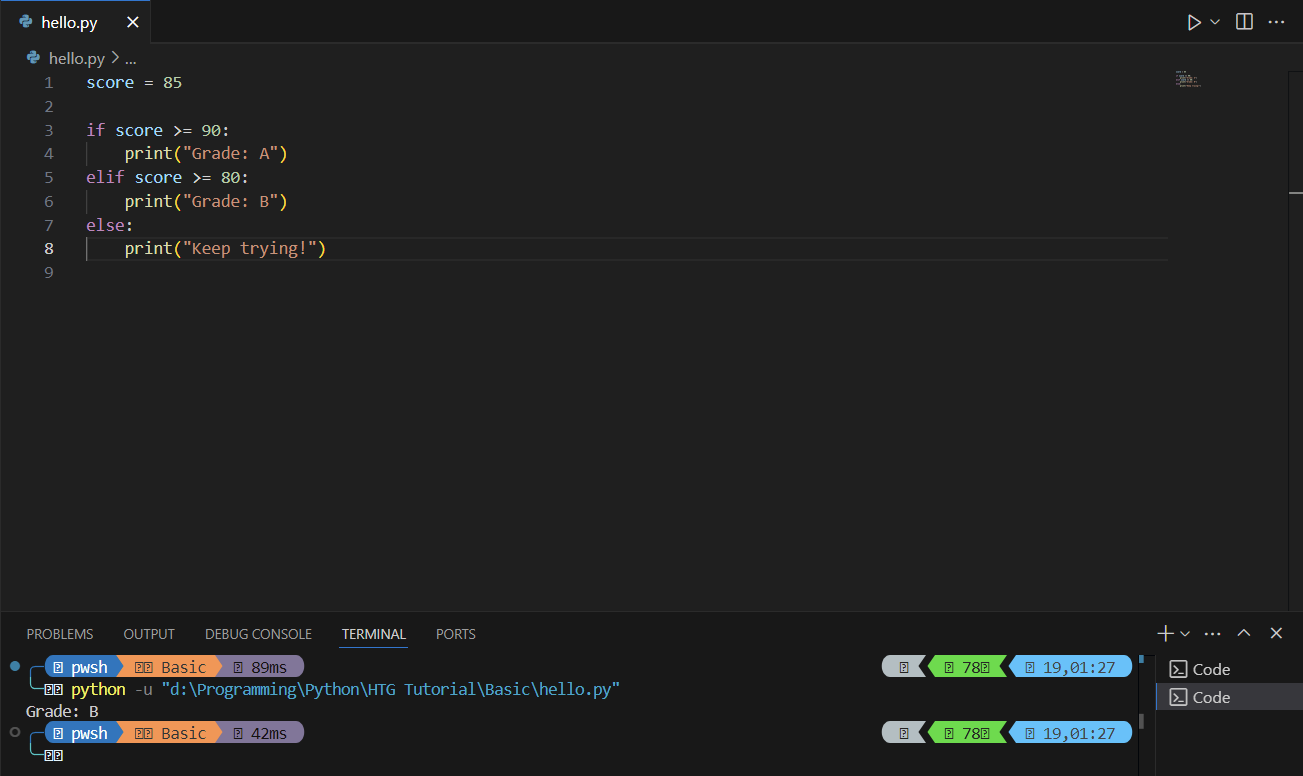
You tin adhd arsenic galore elif conditions arsenic you like.
Loop Through Code with for and while
In programming, we often person to bash repetitive tasks. For specified cases, we usage loops. Instead of copying the aforesaid code, we tin usage loops to bash the aforesaid happening with overmuch little code. We'll larn astir 2 antithetic loops.
for Loop
The for loop is utile erstwhile you privation to tally a artifact of codification a circumstantial fig of times.
for one in range(n):Let's people a substance utilizing a loop.
for one in range(5):print("Hello!")
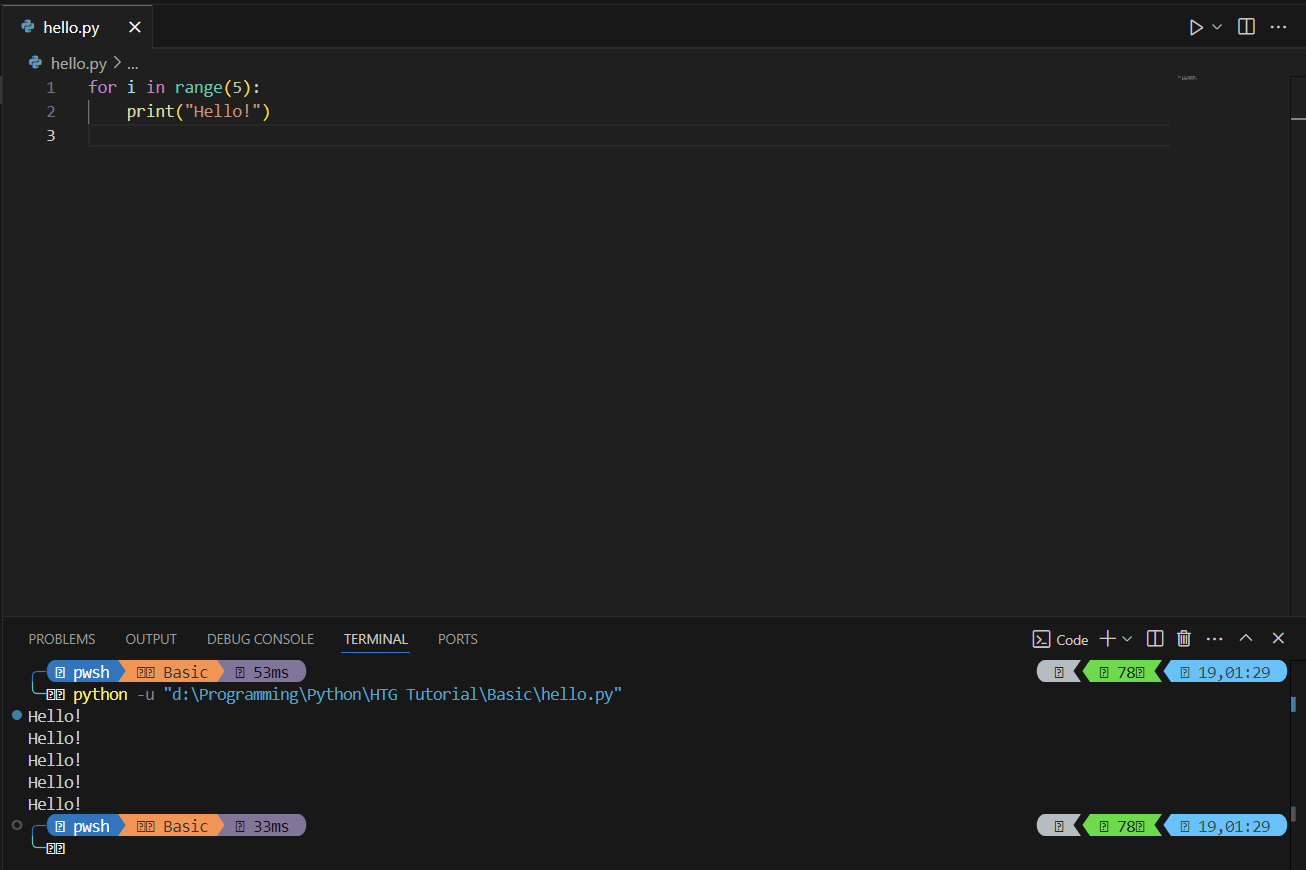
This prints "Hello!" 5 times. The worth of one goes from 0 to 4. You tin besides commencement astatine a antithetic number:
for one in range(1, 4):print(i)
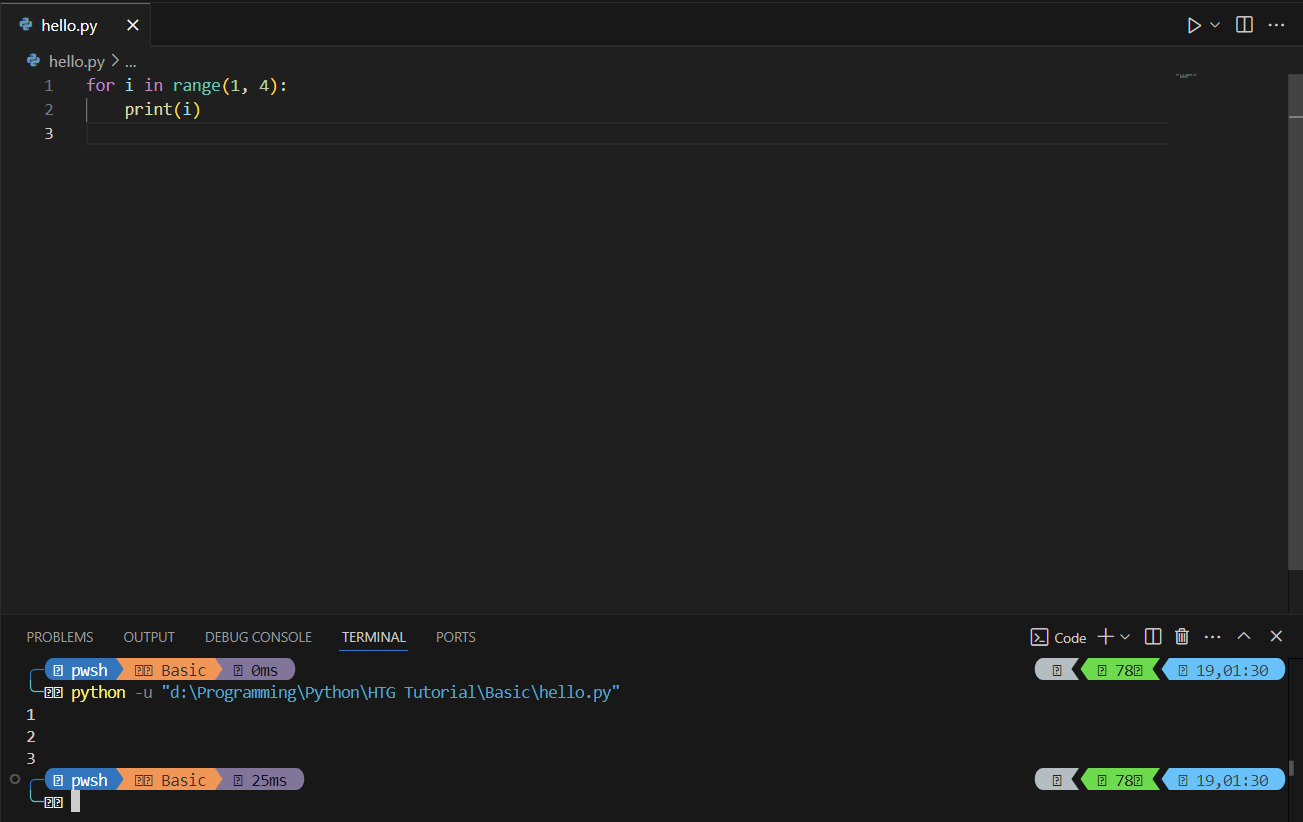
The ending worth (4 successful this case) successful the range() relation isn't printed due to the fact that it's excluded from the range.
portion Loop
The portion loop is utilized erstwhile you're uncertain however agelong the loop volition run. When you privation to support looping arsenic agelong arsenic a information is true.
while condition:A speedy example.
number = 1while number <= 3:
print("Count is", count)
count += 1
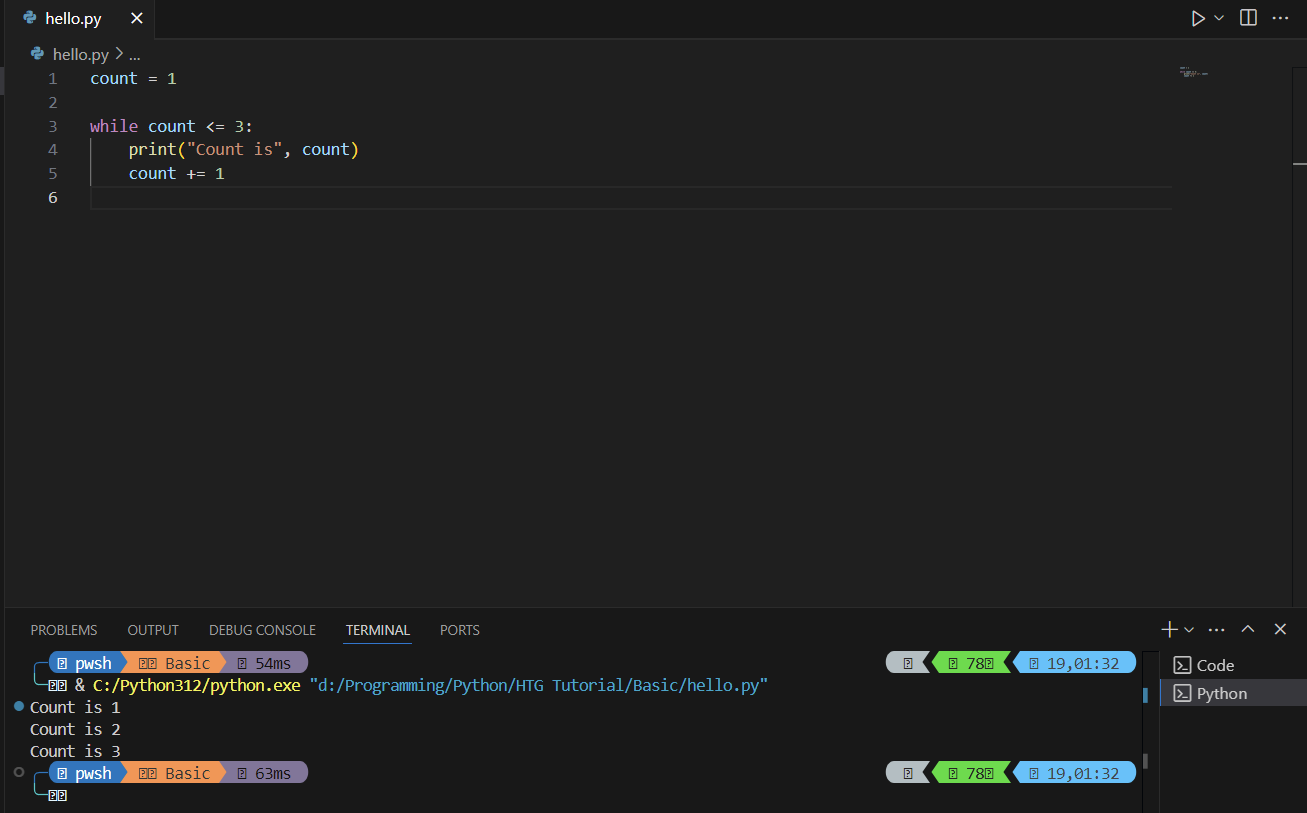
Two much utile statements successful loops are the interruption connection and the proceed statement. The interruption connection stops a loop aboriginal if thing you specify happens.
one = 1while True:
print(i)
if one == 3:
break
i += 1
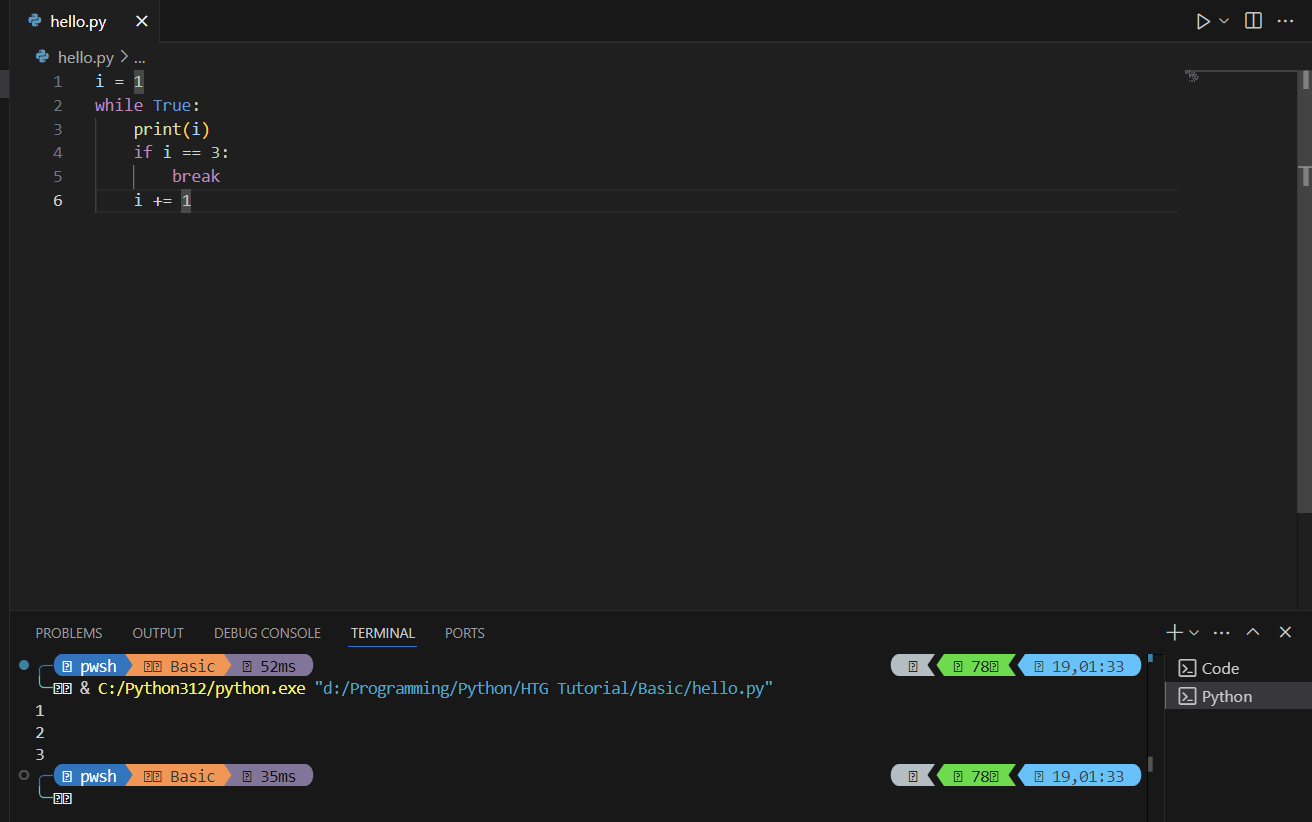
The proceed connection skips the remainder of the codification successful the loop for that iteration, and goes to the adjacent one.
for one in range(5):if one == 2:
continue
print(i)
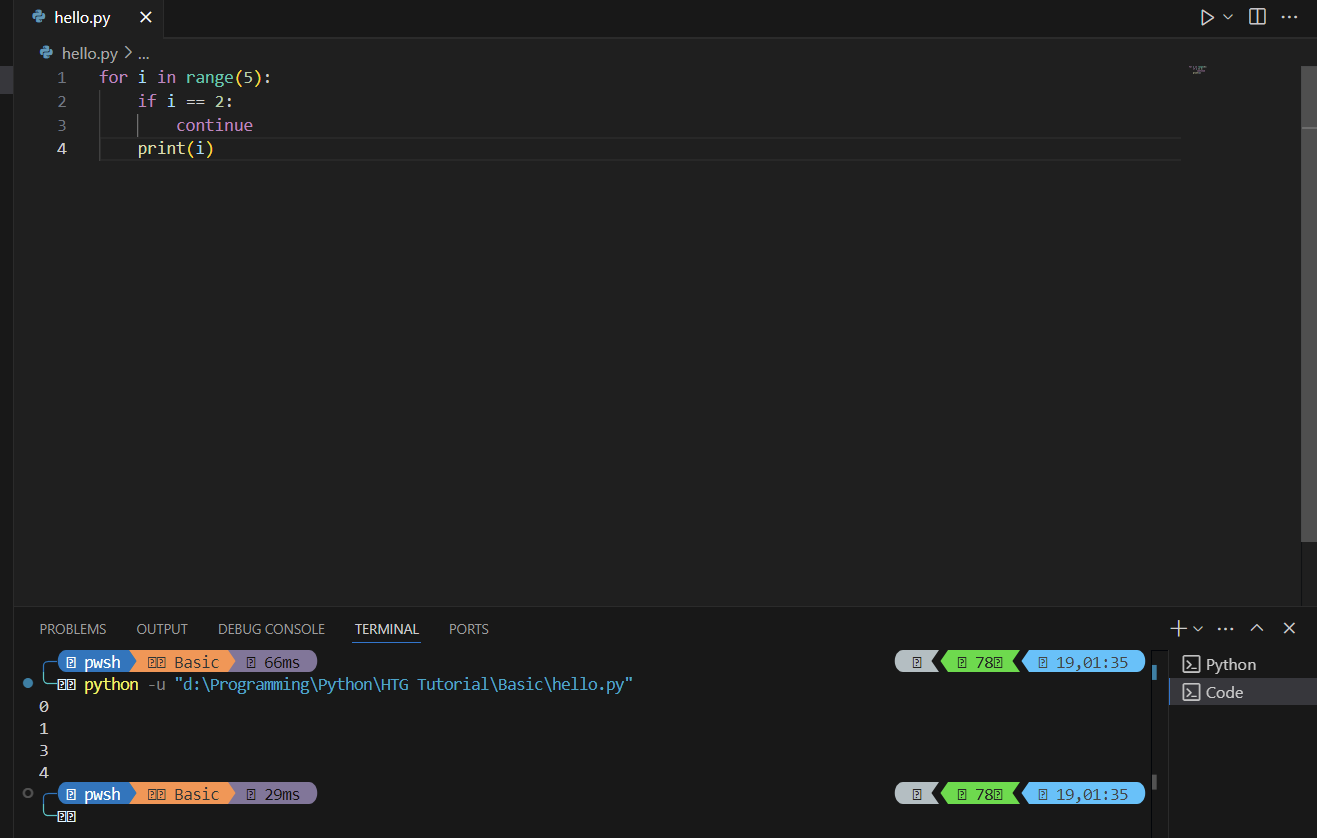
Write Your Own Functions
As your programs get bigger, you'll find yourself typing the aforesaid codification implicit and over. That’s wherever functions travel in. A relation is simply a reusable artifact of codification that you tin "call" whenever you request it. It helps you debar repeating code, signifier your program, and marque codification easier to work and maintain. Python already gives you built-in functions similar print() and input(), but you tin besides constitute your own.
To make a function, usage the def keyword:
def function_name():Then you telephone it similar this:
function_name()Let's make a elemental function:
def say_hello():print("Hello!")
print("Welcome to Python.")
say_hello()
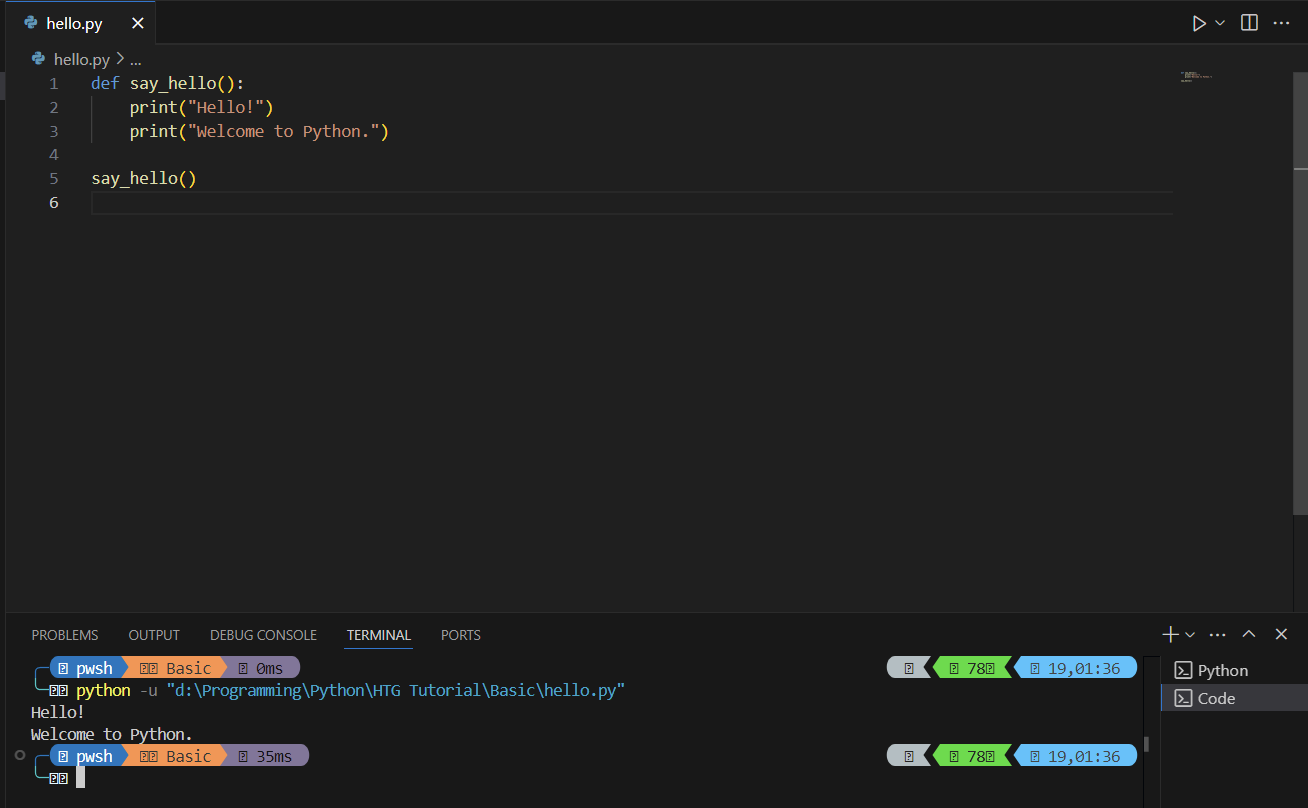
You tin besides marque your relation judge parameters. Parameters are values passed into it.
def greet(name):print("Hello,", name)
To telephone it with an argument:
greet("Alice")greet("Bob")
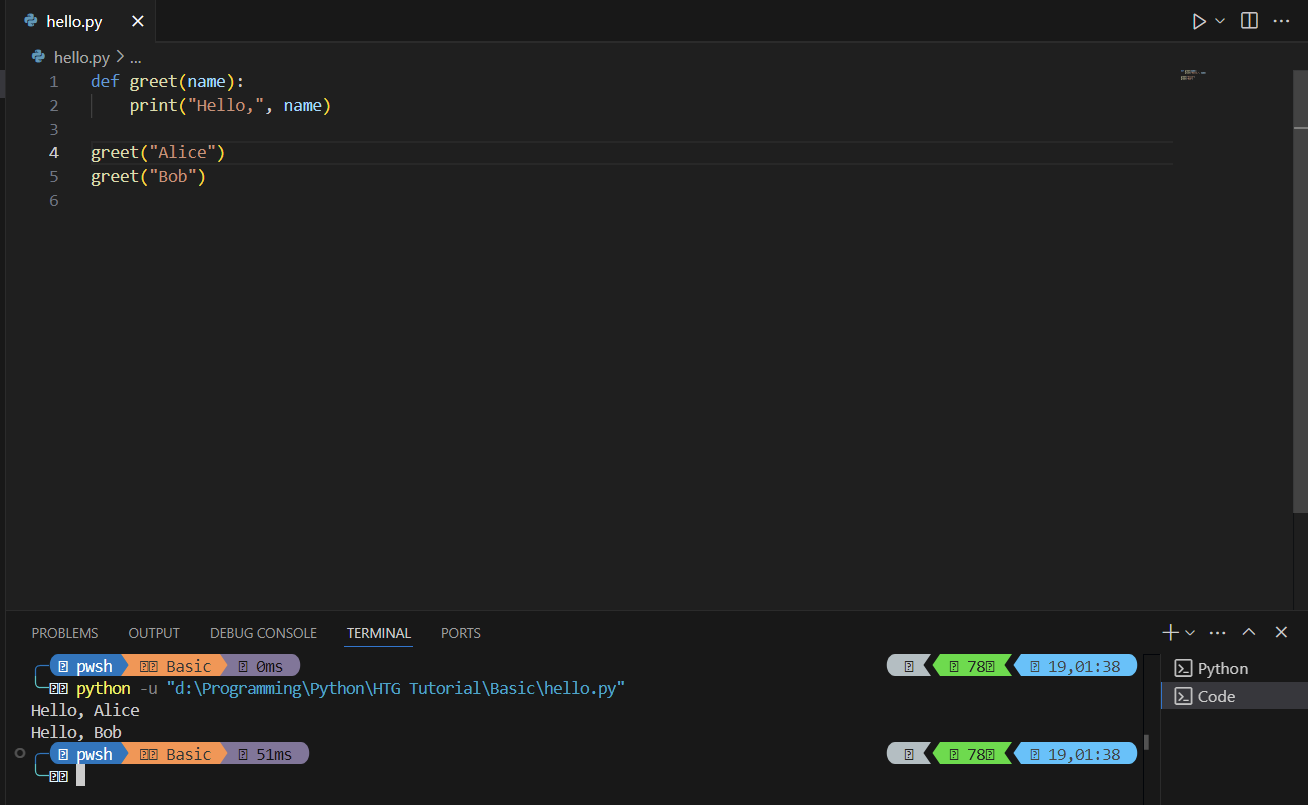
You tin usage aggregate parameters, too.
def add(a, b):print(a + b)
add(3, 4)
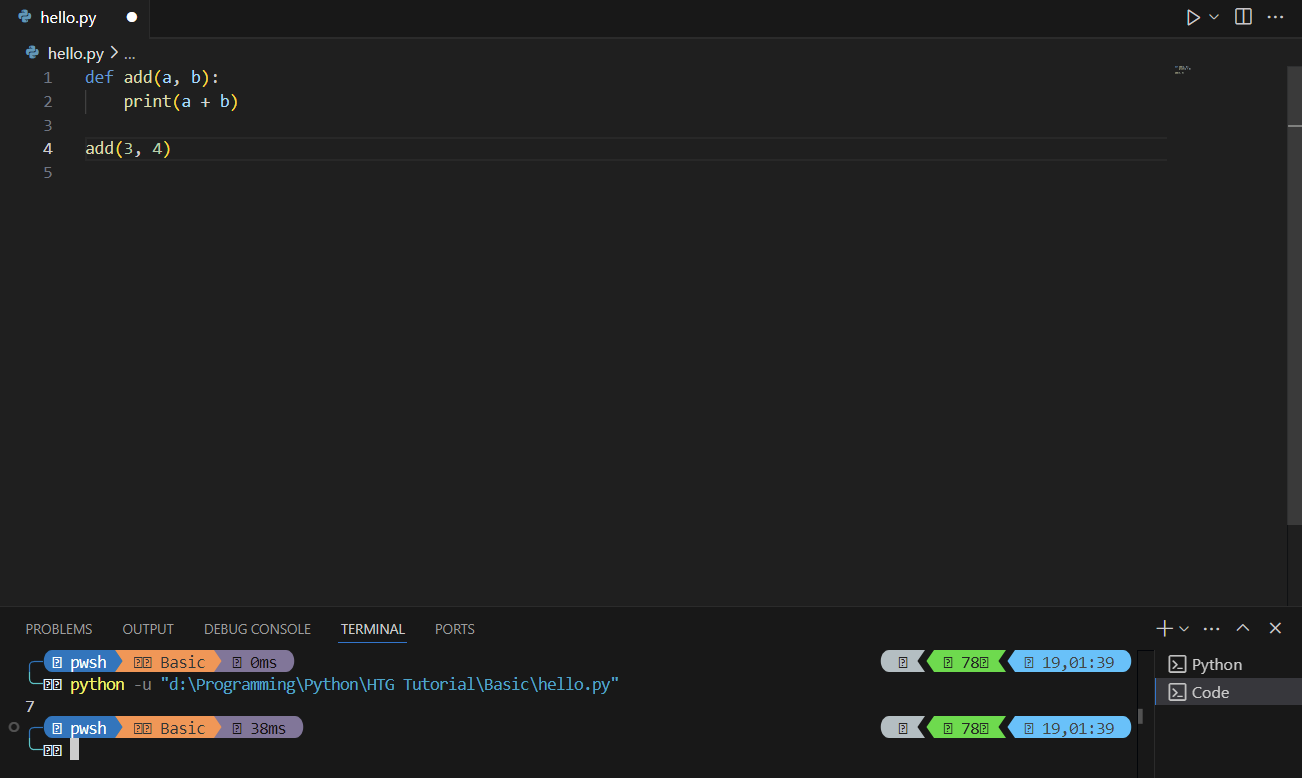
You tin reuse the aforesaid relation aggregate times with antithetic inputs.
That covers immoderate of the basics of Python programming. If you privation to become a amended programmer, you request to commencement doing immoderate projects, specified arsenic an expense tracker oregon a to-do database app.